Imokutr thumbnail library
PHP image thumbnailer created with primary use for Nette framework, but also usable with a standalone PHP apps.
Author: Vladimír Škach
Latest stable version: 1.1.0 (with Nette 3 support) :
Github |
Packagist
Table of content
Basic overview
Let's assume:
1. we have a directory with a bunch of images intended to be visible on our web,
it's absolute path is for example /home/myweb/www/files.
Images can be in JPEG, PNG or GIF format (a transparency is supported).
In configuration we will set it like this:
originalRootPath: /home/myweb/www/files
There is directory structure inside containing images which we are displaying on our web,
their absolute paths are for example:
/home/myweb/www/files/images/2018/06/dog.jpg
/home/myweb/www/files/gallery/25/photo3.png
/home/myweb/www/files/newImage.jpg
On the web these images are visible on these urls:
https://www.myweb.com/files/images/2018/06/dog.jpg
https://www.myweb.com/files/gallery/25/photo3.png
https://www.myweb.com/files/newImage.jpg
2. we also have some kind of data storage, most likely a database, where we store paths to these images,
for example:
id label path
1 Big dog /images/2018/06/dog.jpg
2 Nice scenery /gallery/25/photo3.png
3 Clouds /newImage.jpg
Now, we will specify target directory to which we will be saving the resulting thumbnail.
Let's say it will be /home/myweb/www/thumbnails
So in config we will set both absolute and relative path to this directory:
thumbsRootPath: /home/web/www/thumbnails
thumbsRootRelativePath: /thumbnails
Ikomutr is now able to create thumbnails on demand. We can choose their dimensions or way they will be cut.
Example:
- source path is (/files)/images/2018/06/dog.jpg
- we want Imokutr to create thumbnail:
with fixed width of 200 pixels,
or fixed height of 150 pixels,
or cropped to specific size 200x150 pixels
- Imokutr will create thumbnails and save them to target directory, they will be accessible on respective urls:
https://www.myweb.com/thumbnails/images/2018/06/dog-200x150-1-0.jpg
or https://www.myweb.com/thumbnails/images/2018/06/dog-200x150-2-0.jpg
or https://www.myweb.com/thumbnails/images/2018/06/dog-200x150-3-0.jpg
Quick start with Nette 2.4
Add Imokutr to composer.json
require": {
...,
"skachcz/imokutr": "^1.0"
}
composer update
Add Imokutr extension to config.neon
extensions:
imokutr: SkachCz\Imokutr\Nette\ImokutrExtension
imokutr:
originalRootPath: /home/web/www
thumbsRootPath: /home/web/www/kutr/thumb
thumbsRootRelativePath: /kutr/thumb
defaultImageRelativePath: /kutr/default.png
qualityJpeg: 85
qualityPng: 8
Try macro imoTag in *.latte template:
{imoTag '/images/2018/06/dog.jpg', 150, 150, 'w'}
<img src="%url%" width="%width%" height="%height%" title="Dog">
{/imoTag}
{imoTag '/images/2018/06/dog.jpg', 200, 100, 'c', 1}
<img src="%url%" width="%width%" height="%height%" title="Dog">
{/imoTag}
(%url%, %width%, and %height% placeholders can be used in the macro's body)
Or filter imoUrl:
{$img1|imoUrl:200:100:'h'}
Configuration
originalRootPath
mandatory
absolute filesystem path to root of source images directory repository
thumbsRootRelativePath
mandatory
relative web path to root of target thumbnail directory repository
thumbsRootPath
mandatory
absolute filesystem path to root of target thumbnail directory repository
defaultImageRelativePath
mandatory
relative path to default source image
qualityJpeg
optional, default value 85
quality of created Jpeg images
qualityPng: 8
optional, default value 8
quality of created PNG images
Thumbnail parameters
width
The width of thumbnail in pixels.
height
The height of thumbnail in pixels.
fixed dimension
This parameter specifies how thumbnail is created.
Possible values are: 'w', 'h', 'c'
Examples for width=100 and height=100.
w
Image is resized with defined width.
h
Image is resized with defined height.
c
Image is cropped to defined width and height.
crop type (optional)
How should be image cropped 0-9
↖ ↑ ↗
1 2 3
← 8 0 4 →
7 6 5
↙ ↓ ↘
Standalone use
<?php
use SkachCz\Imokutr\Imokutr;
use SkachCz\Imokutr\Config;
use SkachCz\Imokutr\Html;
require_once(__DIR__ . "/Imokutr/imokutr.php");
$config = new Config(
__DIR__ . "/images/original",
__DIR__ . "/images/thumbs",
"/images/thumbs"
);
$kutr = new Imokutr($config);
// original image is in /images/original/img1.jpg
$img = $kutr->getThumbnail("img1.jpg", 200, 100, 'w');
?>
<img src="<?php echo $img['url'] ?>" width="<?php echo $img['width'] ?>" height="<?php echo $img['height'] ?>">
or
<?php echo Html::img($img) ?>
<?php $img = $kutr->getThumbnail("img1.jpg", 300, 300, 'c', 5); ?>
<?php echo Html::img($img, "Photo", "My photo", ["data-text" => "My text", "onclick" => 'alert("box")', "class" => "red"] ) ?>
Demo
Filter imoUrl:
{'/files/original/docs/kutr/airplane.jpg'|imoUrl:200:100:'h'}
-> /kutr/thumb/files/original/docs/kutr/airplane-200x100-2.jpg
{'/files/original/docs/kutr/airplane.jpg'|imoUrl:200:100:'c':4}
-> /kutr/thumb/files/original/docs/kutr/airplane-200x100-3.jpg
Macro imoTag:
Original images:
Fixed width 200px:
{imoTag '/image.jpg', 200, 200, 'w'}
<img src="%url%" width="%width%" height="%height%" title="titulek">
{/imoTag}
Fixed height 150px:
{imoTag '/image.jpg', 150, 150, 'h'}
<img src="%url%" width="%width%" height="%height%" title="titulek">
{/imoTag}
Cropped 150x150px:
{imoTag '/image.jpg', 150, 150, 'c'}
<img src="%url%" width="%width%" height="%height%" title="titulek">
{/imoTag}
Cropped 150x150px to center (0):
{imoTag '/image.jpg', 150, 150, 'c', 0}
Cropped 150x150px to top left (1):
{imoTag '/image.jpg', 150, 150, 'c', 1}
Cropped 150x150px to right (4):
{imoTag '/image.jpg', 150, 150, 'c', 4}
Cropped 150x150px to bottom left (7):
{imoTag '/image.jpg', 150, 150, 'c', 7}
PHP image thumbnailer created with primary use for Nette framework, but also usable with a standalone PHP apps.
Author: Vladimír Škach
Latest stable version: 1.1.0 (with Nette 3 support) : Github | Packagist
1. we have a directory with a bunch of images intended to be visible on our web, it's absolute path is for example /home/myweb/www/files. Images can be in JPEG, PNG or GIF format (a transparency is supported).
In configuration we will set it like this:
There is directory structure inside containing images which we are displaying on our web, their absolute paths are for example:
On the web these images are visible on these urls:
2. we also have some kind of data storage, most likely a database, where we store paths to these images, for example:
Now, we will specify target directory to which we will be saving the resulting thumbnail.
Let's say it will be /home/myweb/www/thumbnails
So in config we will set both absolute and relative path to this directory:
Ikomutr is now able to create thumbnails on demand. We can choose their dimensions or way they will be cut.
Example:
- source path is (/files)/images/2018/06/dog.jpg
- we want Imokutr to create thumbnail:
with fixed width of 200 pixels,
or fixed height of 150 pixels,
or cropped to specific size 200x150 pixels
- Imokutr will create thumbnails and save them to target directory, they will be accessible on respective urls:
Try macro imoTag in *.latte template:
Or filter imoUrl:
{'/files/original/docs/kutr/airplane.jpg'|imoUrl:200:100:'h'}
-> /kutr/thumb/files/original/docs/kutr/airplane-200x100-2.jpg
{'/files/original/docs/kutr/airplane.jpg'|imoUrl:200:100:'c':4}
-> /kutr/thumb/files/original/docs/kutr/airplane-200x100-3.jpg
Macro imoTag:
Original images:
Author: Vladimír Škach
Latest stable version: 1.1.0 (with Nette 3 support) : Github | Packagist
Table of content
Basic overview
Let's assume:1. we have a directory with a bunch of images intended to be visible on our web, it's absolute path is for example /home/myweb/www/files. Images can be in JPEG, PNG or GIF format (a transparency is supported).
In configuration we will set it like this:
originalRootPath: /home/myweb/www/files
There is directory structure inside containing images which we are displaying on our web, their absolute paths are for example:
/home/myweb/www/files/images/2018/06/dog.jpg /home/myweb/www/files/gallery/25/photo3.png /home/myweb/www/files/newImage.jpg
On the web these images are visible on these urls:
https://www.myweb.com/files/images/2018/06/dog.jpg https://www.myweb.com/files/gallery/25/photo3.png https://www.myweb.com/files/newImage.jpg
2. we also have some kind of data storage, most likely a database, where we store paths to these images, for example:
id | label | path |
1 | Big dog | /images/2018/06/dog.jpg |
2 | Nice scenery | /gallery/25/photo3.png |
3 | Clouds | /newImage.jpg |
Now, we will specify target directory to which we will be saving the resulting thumbnail.
Let's say it will be /home/myweb/www/thumbnails
So in config we will set both absolute and relative path to this directory:
thumbsRootPath: /home/web/www/thumbnails
thumbsRootRelativePath: /thumbnails
Ikomutr is now able to create thumbnails on demand. We can choose their dimensions or way they will be cut.
Example:
- source path is (/files)/images/2018/06/dog.jpg
- we want Imokutr to create thumbnail:
with fixed width of 200 pixels,
or fixed height of 150 pixels,
or cropped to specific size 200x150 pixels
- Imokutr will create thumbnails and save them to target directory, they will be accessible on respective urls:
https://www.myweb.com/thumbnails/images/2018/06/dog-200x150-1-0.jpg or https://www.myweb.com/thumbnails/images/2018/06/dog-200x150-2-0.jpg or https://www.myweb.com/thumbnails/images/2018/06/dog-200x150-3-0.jpg
Quick start with Nette 2.4
Add Imokutr to composer.jsonrequire": {
...,
"skachcz/imokutr": "^1.0"
}
composer update
Add Imokutr extension to config.neon
extensions:
imokutr: SkachCz\Imokutr\Nette\ImokutrExtension
imokutr:
originalRootPath: /home/web/www
thumbsRootPath: /home/web/www/kutr/thumb
thumbsRootRelativePath: /kutr/thumb
defaultImageRelativePath: /kutr/default.png
qualityJpeg: 85
qualityPng: 8
Try macro imoTag in *.latte template:
{imoTag '/images/2018/06/dog.jpg', 150, 150, 'w'}
<img src="%url%" width="%width%" height="%height%" title="Dog">
{/imoTag}
{imoTag '/images/2018/06/dog.jpg', 200, 100, 'c', 1}
<img src="%url%" width="%width%" height="%height%" title="Dog">
{/imoTag}
(%url%, %width%, and %height% placeholders can be used in the macro's body)
Or filter imoUrl:
{$img1|imoUrl:200:100:'h'}
Configuration
originalRootPath | mandatory | absolute filesystem path to root of source images directory repository |
thumbsRootRelativePath | mandatory | relative web path to root of target thumbnail directory repository |
thumbsRootPath | mandatory | absolute filesystem path to root of target thumbnail directory repository |
defaultImageRelativePath | mandatory | relative path to default source image |
qualityJpeg | optional, default value 85 | quality of created Jpeg images |
qualityPng: 8 | optional, default value 8 | quality of created PNG images |
Thumbnail parameters
width | The width of thumbnail in pixels. | ||||||
height | The height of thumbnail in pixels. | ||||||
fixed dimension |
This parameter specifies how thumbnail is created.
Possible values are: 'w', 'h', 'c' Examples for width=100 and height=100.
|
||||||
crop type (optional) |
How should be image cropped 0-9
↖ ↑ ↗ 1 2 3 ← 8 0 4 → 7 6 5 ↙ ↓ ↘ |
Standalone use
<?php
use SkachCz\Imokutr\Imokutr;
use SkachCz\Imokutr\Config;
use SkachCz\Imokutr\Html;
require_once(__DIR__ . "/Imokutr/imokutr.php");
$config = new Config(
__DIR__ . "/images/original",
__DIR__ . "/images/thumbs",
"/images/thumbs"
);
$kutr = new Imokutr($config);
// original image is in /images/original/img1.jpg
$img = $kutr->getThumbnail("img1.jpg", 200, 100, 'w');
?>
<img src="<?php echo $img['url'] ?>" width="<?php echo $img['width'] ?>" height="<?php echo $img['height'] ?>">
or
<?php echo Html::img($img) ?>
<?php $img = $kutr->getThumbnail("img1.jpg", 300, 300, 'c', 5); ?>
<?php echo Html::img($img, "Photo", "My photo", ["data-text" => "My text", "onclick" => 'alert("box")', "class" => "red"] ) ?>
Demo
Filter imoUrl:{'/files/original/docs/kutr/airplane.jpg'|imoUrl:200:100:'h'}
-> /kutr/thumb/files/original/docs/kutr/airplane-200x100-2.jpg
{'/files/original/docs/kutr/airplane.jpg'|imoUrl:200:100:'c':4}
-> /kutr/thumb/files/original/docs/kutr/airplane-200x100-3.jpg
Macro imoTag:
Original images:
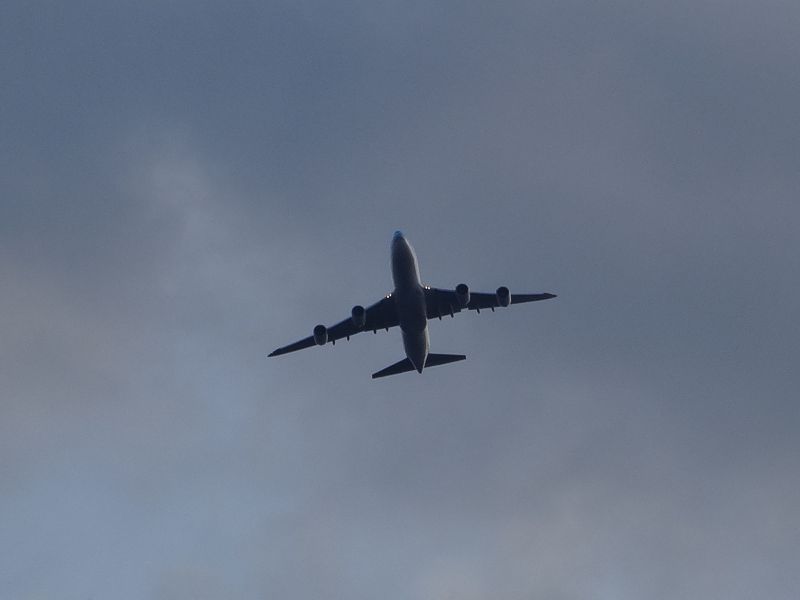
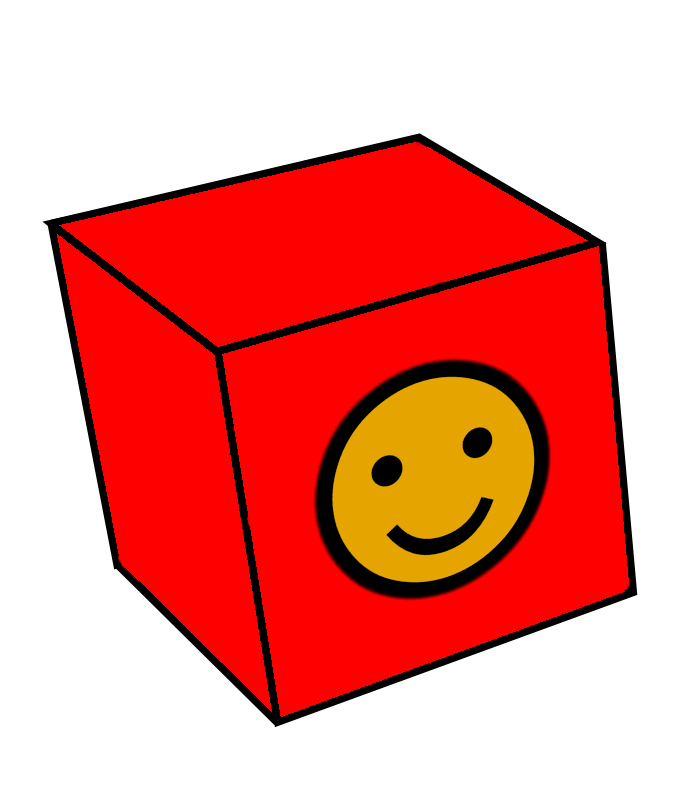
Fixed width 200px:
{imoTag '/image.jpg', 200, 200, 'w'}
<img src="%url%" width="%width%" height="%height%" title="titulek"> {/imoTag} ![]() ![]() |
Fixed height 150px:
{imoTag '/image.jpg', 150, 150, 'h'}
<img src="%url%" width="%width%" height="%height%" title="titulek"> {/imoTag} ![]() ![]()
Cropped 150x150px:
{imoTag '/image.jpg', 150, 150, 'c'}
<img src="%url%" width="%width%" height="%height%" title="titulek"> {/imoTag} ![]() ![]() |
Cropped 150x150px to center (0):
{imoTag '/image.jpg', 150, 150, 'c', 0}
![]() ![]()
Cropped 150x150px to top left (1):
{imoTag '/image.jpg', 150, 150, 'c', 1}
![]() ![]()
Cropped 150x150px to right (4):
{imoTag '/image.jpg', 150, 150, 'c', 4}
![]() ![]()
Cropped 150x150px to bottom left (7):
{imoTag '/image.jpg', 150, 150, 'c', 7}
![]() ![]() |